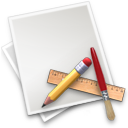
It’s time for the last, 3rd part of basic Prototype JS course, where we focus on the use of forms, associative arrays, strings and others, but this time on the rules of Prototype JS.
Further delving into the Prototype JS framework
Let’s start with the forms.
Form
Prototype JS encapsulates and / or extends to us many aspects of programming, including forms support.
Available methods:
– disable() – deactivate element, for example:
form[form.disabled ? 'enable' : 'disable'](); form.disabled = !form.disabled;
– enable() – activate the element
– findFirstElement(formElement) – find the first element
– focusFirstElement() – go to the first element (item)
– getElements() – get all elements of the form
– getInputs() – get input fields, for example:
var form = $('myform'); form.getInputs(); // -> all INPUT elements form.getInputs('text'); // -> all text inputs // only radio buttons with the name "education" var buttons = form.getInputs('radio', 'education'); // set disable = true for these buttons buttons.invoke('disable');
– request() – automatically creates the Ajax.Request, where the parameters are built on the basis of form fields, e.g.:
<form id="person-example" method="POST" action="file.php"> … $('person-example').request(); // sending // add a callback: $('person-example').request({ onComplete: function(){ alert('Form data saved!') } }) // get $('person-example').request({ method: 'get', parameters: { interests: 'JavaScript', 'hobbies[]' : ['programming', 'music'] }, onComplete: function(){ alert('Form data saved!') } })
– reset() – reset of the form fields
– serialize() – serializes form fields — as keys (names) and the fields values, for example:
// Test 1 // 'username=tester&age=22&hobbies=coding' $('person-example').serialize(); // Test 2 // {username: 'tester', age: '22', hobbies: ['coding', 'JS']} $('person-example').serialize(true);
– serializeElements() – serializes elements, for example:
new Ajax.Request('/some_url', { parameters: $('id_of_form_element').serialize(true) });
Form.element
Now we will take care about operations on the form fields, including getting their values.
The most important methods:
– argumentNames() – returns names of arguments, e.g.:
var fn = function(foo, bar) { return foo + bar; }; fn.argumentNames(); // -> ['foo', 'bar']
– bind() – associate with the function, e.g.:
var obj = { name: 'A nice demo', fx: function() { alert(this.name + '\n' + $A(arguments).join(', ')); } }; var fx2 = obj.fx.bind(obj, 1, 2, 3); fx2(4, 5); // alert: the proper name, then "1, 2, 3, 4, 5"
– bindAsEventListener() – associate with the Event Listener (listening and handling events), for example:
var obj = { name: 'A nice demo' }; function handler(e) { var tag = Event.element(e).tagName.toLowerCase(); var data = $A(arguments); data.shift(); alert(this.name + '\nClick on a ' + tag + '\nOther args: ' + data.join(', ')); } Event.observe(document.body, 'click', handler. bindAsEventListener(obj, 1, 2, 3));
Example #2 — monitoring of the elements of the specified type (checkbox watcher):
var CheckboxWatcher = Class.create(); CheckboxWatcher.prototype = { initialize: function(chkBox, message) { this.chkBox = $(chkBox); this.message = message; this.chkBox.onclick = this.showMessage.bindAsEventListener(this, ' from checkbox'); }, showMessage: function(evt, extraInfo) { alert(this.message + ' (' + evt.type + ')' + extraInfo); } }; var watcher = new CheckboxWatcher('myChk', 'Changed');
Hash — associative arrays support
Another topic, where Prototype JS helps the programmer in work with the associative arrays (sometimes they are called “hash”).
We will show how it looks in the newer versions of the API.
Sample code:
var myhash = new Hash(); myhash.set('name', 'Bob'); myhash.get('name'); myhash.unset('name'); // …
The methods available for objects of Hash type:
– clone() – cloning, in the same way as for ordinary tables
– each() – iterating through the elements, e.g.:
var h = $H({ version: 1.5, author: 'Steve Stephenson' }); h.each(function(pair) { alert(pair.key + ' = "' + pair.value + '"'); });
– get() – gets the value from a given key, for example:
var h = new Hash({ a: 'apple', b: 'banana', c: 'coconut' }); h.get('a');
– inspect() – inspection; useful among others for debugging
– keys() – returns keys, e.g.:
$H({ name: 'Prototype', version: 1.5 }).keys().sort(); // ['name', 'version']
– merge() – merging
– remove() – removing elements, e.g.:
var h = new Hash({ a: 'apple', b: 'banana', c: 'coconut' }) h.remove('a', 'c'); // ['apple', 'coconut']
– set() – settings elements (items)
// if an index exists, then will be overwritten! h.set('d', 'orange');
– toJSON() – converts hash (an associative array) to the JSON format
– toObject() – conversion to an object, for example:
var h = new Hash({ a: 'apple', b: 'banana', c: 'coconut' }); var obj = h.toObject();
– toQueryString() – conversion to the HTTP query string, e.g.:
$H({ action: 'ship', order_id: 123, fees: ['f1', 'f2'], 'label': 'a demo' }).toQueryString(); // -> 'action=ship&order_id=123&fees=f1&fees=f2&label=a%20demo'
– unset() – delete the item, e.g.:
h.unset('a');
– update() – updating an item, e.g.:
var h = $H({ name: 'Prototype', version: 1.5 }); h.update({ version: 1.6, author: 'Sam' }).inspect();
– values() – getting values (see also: “keys”), for example:
$H({ name: 'Prototype', version: 1.5 }). values().sort(); // [1.5, 'Prototype']
If we will work with associative arrays in JS, we will appreciate the toolbox given us by the Prototype JS framework.
Now let’s take a look how to work with the DOM in Prototype JS.
Insertion — inserting elements into the document
So an attempt to improve the DOM handling. We obtain the following methods:
After() – insert after the given element
Before() – insert before the given element
Bottom() – insert at the end of the collection
Top() – insert at the beginning of the collection
Example:
<div><p id="foobar">Some text…</p></div>
new Insertion.After('foobar', "<span>Something new …</span>");
It is often used together with the AJAX operations, for programming dynamic updates of the document.
Strings
So Prototype JS and impressive capabilities of work with strings.
Methods:
– blank() – checks if a string is empty, e.g.:
' '.blank(); // true
– camelize() – returns the “Camel Case” string, for example:
'background-color'.camelize(); // 'backgroundColor' '-moz-binding'.camelize(); // 'MozBinding'
– capitalize() – the first letter big, other letters small
– dasherize() – changing _ to -, e.g.:
'border_bottom'.dasherize(); // 'border-bottom'
– empty() – checks if a string is empty, e.g.:
- ' '.empty(); // false
– endsWith() – checks whether a string ends with a substring, for example:
'biglol'.endsWith('lol'); // true
– escapeHTML() – safe HTML — replace tags for entities,e.g.:
'<div>This is an article</div>'.escapeHTML(); // -> "&lt;div&gt;This is an article&lt;/div&gt;"
– evalJSON([sanitize = false]) – JSON object code execution, sanitize = true should be used
– evalScripts() – execute the JS code given in the string
'lorem… <script>2 + 2</script>'.evalScripts(); // [4]
– extractScripts() – extract the code from the script tags:
'<script>2+2</script><script>var i = 5</script>'.extractScripts(); // ['2+2', 'var i = 5']
– gsub() – returns a string, where each occurrence of the pattern has been replaced
– include() – checks whether the string contains a substring
– inspect() – inspection; creates the debug-oriented string
– interpolate() – interpolation of values; see also: Template
– isJSON() – checks whether we are dealing with JSON, for example:
"{ \"foo\": 42 }".isJSON() // true
– parseQuery() – toQueryParams alias
– scan(pattern, iterator) – scan for a pattern, for example:
'apple, pear & orange'.scan(/\w+/, alert); // 'apple pear orange'
– startsWith() – checks whether a string starts with a substring, for example:
'PrototypeJS'.startsWith('Pro'); // true
– strip() – removes breaks / whitespace
– stripScripts() – removes everything what looks like a script
– stripTags() – removes HTML tags
– sub(pattern, replacement[, count = 1]) – simplification of the substring method
– succ() – changes the character to the next (according to Unicode), such as:
'a'.succ(); // 'b' 'aaaa'.succ(); // 'aaab'
– times(N) – repeat string N times
– toArray() – convert a string to an array
– toJSON() – conversion to JSON
– toQueryParams() – creates an object based on the URI, such as:
'http://www.test.com?section=blog&id=48#comments'.toQueryParams(); // {section: 'blog', id: '48'} 'tag=ruby%20on%20rails'.toQueryParams(); // {tag: 'ruby on rails'}
– truncate([length = 30[, suffix = ‘…’]]) – cut the string according to parameters, e.g.:
'Some random text'.truncate(10); // 'Some ra…' 'Some random text'.truncate(10, ' […]'); // 'Some […]'
– underscore() – make words separated (by underscore) in the given “Camelcase” string
'borderBottomWidth'.underscore(); // 'border_bottom_width' 'borderBottomWidth'.underscore().dasherize(); // 'border-bottom-width'
– unescapeHTML() – replace entities with tags, see also: escapeHTML(); example:
'x > 10'.unescapeHTML(); // 'x > 10'
– unfilterJSON() – removes comment delimiters from Ajax response
Now let’s go to handling the Document object.
Document
Prototype JS also extends the Document object.
A simple example:
document.observe("dom:loaded", function() { $$('div.tabcontent').invoke('hide'); });
There is also Document.viewport available (screen data).
Examples:
document.viewport.getDimensions(); // e.g { width: 776, height: 580 } document.viewport.getHeight(); document.viewport.getWidth(); document.viewport.getScrollOffsets(); // e.g { left: 0, top: 0 } document.viewport.getScrollOffsets(); // e.g { left: 0, top: 120 }
Thus, we are approaching the end of the basic course.
Sample code — OOP, DOM and Ajax with Prototype JS:
// scores publication var ScoreBroadcaster = { setup: function() { this.executer = new PeriodicalExecuter( this.update.bind(this), 30); this.update(); }, update: function() { this.request = new Ajax.Request("scores.php", { onSuccess: this.success.bind(this) }); }, success: function(request) { document.fire("score:updated", request.responseJSON); } }; document.observe("dom:loaded", function() { ScoreBroadcaster.setup(); }); // nasłuchiwanie wyników document.observe("dom:loaded", function() { document.observe("score:updated", function(event) { alert(event.memo); // lub // console.log("received data: ", event.memo); }); });
Summary
And it’s all in the “Prototype JS in a nutshell” series. But in the future we will present more examples or ready scripts based on this library.
Have fun with Prototype JS!