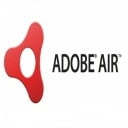
Previously we talked about Adobe AIR basics, including preparation of development environment. It’s time for the next article, and more practice.
We will work with windows and XHR calls (yes, we can enjoy AJAX in AIR apps).
Working with windows in Adobe AIR
Creating a window looks exactly the same as it is in classic JavaScript.
Example — creating a window in Adobe AIR:
function createWindow() { window.open("content.html", "MyWin", "height=300, width=300"); } createWindow();
Adobe AIR goes a step further, giving us the Native Windows, with customization possibility.
Example:
function createNativeWindow() { // windows options var options = new air.NativeWindowInitOptions(); // the window size and position: var rect = new air.Rectangle(20, 20, 300, 250); // create a new window: popup = air.HTMLLoader.createRootWindow(true, options, false, rect); // load the content: var page = air.File.applicationDirectory .resolvePath('content.html'); popup.load(new air.URLRequest(page.url)); } createNativeWindow();
We can customize the window options using dedicated methods; this example shows how to read the contents of an external file (content.html).
By the way, we can see how the reference to file system in AIR looks:
var page = air.File.applicationDirectory .resolvePath('content.html'); popup.load(new air.URLRequest(page.url));
Customizing buttons of the window
One of many possibilities is to define our own window control buttons (min, max, close).
Example:
CSS
.button { position: absolute; top: 5px; font-family: monospace; color: #900; font-size: 12px; } #close { width: 80px; right: 0px; } #min { width: 40px; right: 80px; } #max { width: 40px; right: 120px; }
HTML
<button id="close" onclick="win.close();">Close</button> <button id="min" onclick="win.minimize();">Min</button> <button id="max" onclick="win.maximize();">Max</button>
Additionally we can also handle dragging and resizing windows, by adding the elements as shown below:
<span id="move" onmousedown="win.startMove();">Move</span> <span id="resize" onmousedown="win.startResize (air.NativeWindowResize.BOTTOM_RIGHT);"> Size</span>
Creating menus in AIR applications
This task is as simple as creating windows.
Generally we define functions for handling menu, e.g.:
function doExit(e) { air.NativeApplication.nativeApplication.exit(); }
Then we create a base menu:
var rootMenu = new air.NativeMenu();
and sub-menu:
var fileMenu = new air.NativeMenu();
and then we add the item with the name of the handler to the sub-menu:
var exit = new air.NativeMenuItem('Exit'); exit.addEventListener(air.Event.SELECT, doExit); fileMenu.addItem(exit);
Sources of this example as Aptana project, you can download here.
In this sample project we can find also examples of shortcuts definition, and some OS-related issues.
XMLHttpRequest (XHR)
Adobe AIR has the advantage that we can use things already developed for JavaScript.
So we can even try to migrate codes developed for other projects, and to use well-known jQuery or AJAX technology in general.
And that illustrates the next example — XHR requests execution in Adobe AIR:
var xmlhttp; function appLoad() { var url = "https://javascript-html5-tutorial.com/"; xmlhttp = new XMLHttpRequest(); xmlhttp.open("GET", url, true); xmlhttp.onreadystatechange = function() { if (xmlhttp.readyState == 4) { alert(xmlhttp.responseText); } } xmlhttp.send(null) }
It should look familiar.
Summary
We talked about handling windows, menus and XHR. This broadens our understanding of the opportunities offered by Adobe AIR.
Thank you and enjoy your AIR development.