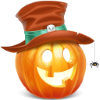
I love this atmosphere of Halloween! Having a free moment, I decided to create a simple game to fully feel the atmosphere.
Halloween game in JavaScript
It’s about the time not complicated requirements. For the project (and writing about on this blog) I had not too much time. Therefore, the aim was to create a game ASAP.
So in the shortest path, we’ll create a simple and well-known Memory game. Matched pictures disappear revealing a stunning background 🙂
The result looks like this:
So let’s code!
The memory game in JavaScript especially for Halloween
1. Nice graphic is the key
But we don’t have a time to create pictures. There would be great to play some scary sounds in background, bot for the moment we skip this topic.
We will use gfx available for FREE. I gathered icons from http://iconfinder.com/. Then adjusted their size (100 x 100 px). In our case we need 10 icons (20 fields on the board).
I also found a nice picture for the background. We have Halloween resources, so go to work.
2. The code
What we need is to revive these resources (like zombies :-)).
The code we will create in HTML5 and jQuery. Even in such a simple game we have to do couple complicated operations.
We save the time, so it’s good idea to take a look on Internet resources (e.g. on GitHub), to avoid writing everything from scratch. I have found a nice example on codepen.io, which can help to make it faster.
3. Create UI / style
We need to define CSS styles for the background, board and buttons, among others:
… body { background-image: url('../gfx/bg2.jpg'); color: #fff; font: 20px Verdana; font-weight: bold; text-transform: uppercase; } #gameBoard { margin: 0px auto; width: 640px; } #boxes { margin: 10px 0 0; z-index: 1; } …
And also the HTML structure:
… <div id="gameBoard"> <span id="gameButtons"> <span class="button"> <span id="counter">0</span> Moves </span> <span class="button"> <a onclick="startAgain();">Start again</a> </span> </span> <div id="boxes"> </div> </div> …
It’s time for JavaScript! BTW even Aptana has Halloween colors 🙂
In the JavaScript code we need a board, counter and images. The names of images are not important — we use them as elements of an array:
var mainBoard = "#boxes"; var counter = 0; … var gfxBase = [ "gfx/icon1.png", "gfx/icon2.png", … "gfx/icon9.png", "gfx/icon10.png" ]; // preload images $(gfxBase).each(function() { var image = $('<img />').attr('src', this); }); …
Pictures must be placed randomly, so we add two functions:
… function doRandom(max, min) { return Math.round(Math.random() * (max - min) + min); } // put images in random order function shuffleImgs() { … } …
The UI contains the “Start again” button, which resets a whole game. Here is the handler:
function startAgain() { shuffleImgs(); $(mainBoard + " div img").hide(); $(mainBoard + " div").css("visibility", "visible"); $("#success").remove(); counter = 0; $("#counter").html("" + counter); cardOpened = ""; imageOpened = ""; imgFound = 0; return false; }
The main point of the code is the function:
function openCard() { … }
In this function whole management is done: show / hide cards with animations, counter, checking for matchings and the game over state:
… if (imgFound == gfxBase.length) { $("#counter").prepend('<span id="success">Done! With </span>'); // alert('BOOOO!'); } …
At the end we run everything — building the board and drawing random pictures:
$(function() { for (var y = 1; y < 3 ; y++) { $.each(gfxBase, function(i, val) { $(mainBoard).append("<div id=card" + y + i + "> <img src=" + val + " />"); }); } $(mainBoard + " div").click(openCard); shuffleImgs(); });
And that’s it. We can play our Halloween game!
The game on-line available here:
Full sources on GitHub:
https://github.com/dominik-w/js_html5_com/tree/master/halloween-memory-game
Summary
Halloween is cool! And in a few moments, we wrote a small, quite nice looking game, related with Halloween. Simple, because there was no time.
Would be great to add some animated elements in the background, or the sound. However, for a few moments spent on the project, we have quite a nice base for development.
Booooo!
Great short tutorial, I am using this to teach myself a bit of JavaScript now. Plus I discovered a great editor for JavaScript: Brackets that updates the webpage you build live as you enter the code — how cool is that? I was learning Java but this is so much more cool!
[…] https://javascript-html5-tutorial.com/a-simple-halloween-game-in-javascript-rapid-development-with-jquery.html; […]
BTW it’s good to fix the small detail of background image…
from:
> background-image: url(‘../gfx/bg2.jpg’);
to:
> background: #000 url(‘../gfx/bg2.jpg’) no-repeat center center fixed;
Cheers!