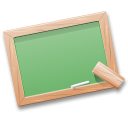
Today, the second part of exploring the Backbone.js secrets.
Backbone.js tutorial
After talking about the basics and key aspects, such as models and collections, we will focus on next — Router, View and Events.
Routing — Backbone.Router
For what would be our application without the possibility of interaction?
Backbone.Router provides routing methods for client-side pages, connections with actions and events. There is also “graceful fallback” solution for browsers without History API support.
Example:
MyRouter = Backbone.Router.extend({ routes: {'hello' : 'sayHello'}, sayHello: function() { console.log('Saying hello'); } }); var router = new MyRouter(); Backbone.history.start();
So we simply define a route with associated function to execute, and then we initialize the Backbone.history:
Backbone.history.start();
That’s it — we can test our code.
To do this, let’s add in URL the name of our “route”, e.g. #hello:
http://url/index.html#hello
As a base, you can use sample code from the previous part:
https://javascript-html5-tutorial.com/backbone-js-tutorial-for-beginners-part-12.html
We can expand the definitions and create a kind of “menu”. We can also define routing parameters, like in following example:
var Workspace = Backbone.Router.extend({ routes: { "help": "help", // #help "search/:query": "search", // #search/cars "search/:query/p:page": "search" // #search/cars/p7 }, help: function() { … }, search: function(query, page) { … } });
Views — Backbone.View
Views are rather a convention used in Backbone. The general idea is the logical organization of the interface — views that can immediately respond to changes in the models, without reloading the page.
SearchView = Backbone.View.extend({ initialize: function() { alert("Hey!"); } }); var search_view = new SearchView();
This is a great place to use templates (JavaScript templating).
The “el” property refers to the DOM object created in the browser, and is an element associated with the view.
Each view in Backbone.js has the “el” property (if not defined, Backbone.js will create his own — empty div element).
Let’s see this in example:
<div id="search_container"></div>
SearchView = Backbone.View.extend({ initialize: function() { alert("Hey"); } }); var search_view = new SearchView({ el: $("#search_container") });
Loading the template
As we know, Backbone.js is dependent on Underscore.js, which offers its own solution for templates (micro-templating).
Let’s write simple function render(), that will call and load the template to the element defined by “el” of our view, using jQuery.
A full example
HTML — the template and container element
<script type="text/template" id="search_template"> <label>Search</label> <input type="text" id="search_input" /> <input type="button" id="search_button" value="Search" /> </script> <div id="search_container"></div> <script type="text/javascript"> SearchView = Backbone.View.extend({ initialize: function() { this.render(); }, render: function() { // compile the template using underscore var template = _.template($("#search_template").html(), {}); // load the compiled HTML into the Backbone "el" this.$el.html(template); } }); var search_view = new SearchView({ el: $("#search_container") }); </script>
I enclose additional examples (from the book Beginning Backbone.js) of using templates:
http://directcode.eu/samples/backbonejs-tutorial-002-templates.zip
OK, it’s time to say something about handling events.
Backbone.js — Events
Events can be added to any object. Backbone event module allows us to bind and trigger built-in events, and also our custom events.
Binding Events
Binding means, that the object is configured to listen for event.
In Backbone.js we do it using function .on(). It accepts three parameters: event name, function to execute in case of event, and optionally — the context.
Example – listen for changes of the author attribute:
model.on("change:author", function() { console.log('The author attribute has been changed'); });
Unbinding Events
Disconnecting the event handler can be done in a simple way, thanks to .off() function:
ourObject.off('change:author');
Listening once? No problem, in Backbone we can define the “one-time” event handler:
model.once("change:author", function() { … });
Everything happens automatically, so we don’t have to unbind the event.
Resources
– Backbone.js website:
– a set of Backbone.js tutorials:
I also recommend the book: Beginning Backbone.js (Apress).
A word about Marionette
Backbone.Marionette is a solution designed to facilitate the construction of large-scale applications.
Project homepage:
Or maybe Chaplin?
This is another solution to use with Backbone.js, supporting the programmers in building scalable, single-page applications, by providing appropriate architecture and best practices.
Project homepage:
Summary
And here we finish our two-piece Backbone.js tutorial for beginners. But as we work more and more with Backbone, we will also write more about this framework in the future.
Thank you!