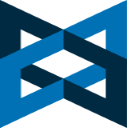
Backbone.js is popular JavaScript library, used heavy to create single-page web applications.
The library was constructed using RESTful JSON interface and model–view–presenter (MVP) pattern. The creator of Backbone.js is Jeremy Ashkenas — author of underscore.js and CoffeeScript.
Backbone.js tutorial for beginners
Backbone.js needs only one dependency to work: Underscore.js (>= 1.5.0).
Example – working with Backbone.js
var object = {}; _.extend(object, Backbone.Events); object.on("alert", function(msg) { alert("Triggered " + msg); }); object.trigger("alert", "an event");
Library introduces us to another level of abstraction. When working with Backbone, we can stop looking at our Web application from JavaScript DOM point of view.
With Backbone.js we represent our data as a model, that can be created, verified (validation), deleted, or saved on the server.
Backbone.js has four fundamental concepts (layers):
– Backbone.Model
– Backbone.View
– Backbone.Router
– Backbone.Collection.
Models — Backbone.Model
The application start here. The model is a layer needed to manipulate data (and representation in the application).
Example – using model:
var artist = new Backbone.Model({ firstName: "John", lastName: "Doe III" }); artist.set({birthday: "December 14, 1987"}); alert(JSON.stringify(artist));
Example – construct a model with default values:
Book = Backbone.Model.extend({ initialize: function() { console.log('Creating new book'); }, defaults: { name: 'JavaScript Book', author: 'Me' } });
To start the examples we have to include necessary libraries. To make things faster, basic template of application you can download here:
http://directcode.eu/samples/backbonejs-tutorial-001.zip
Some events are displayed as alerts, other in console (e.g. of Firebug).
Getting attribute values
Attributes from the model object can be retrieved in a simple manner: .get(“attr_name”).
Example:
var myBook = new Book(); alert(myBook.get('name')); alert(myBook.get('author')); alert(myBook.get('dummy') || 'No attribute');
Removing attributes
When we need to remove unnecessary attribute from model, we use the .unset(“attr_name”) function.
… myBook.unset('name'); alert(myBook.get('name')); // undefined …
Model clone
We can clone our model also in a simple manner:
myBook.clone();
Adding functions — extending the model
The model can be also extended by the functions, that operate on data and perform other operations.
Book = Backbone.Model.extend({ initialize: function() { console.log('Creating new book'); }, defaults: { name: 'JavaScript Book', author: 'Me', when: '2014' }, showInfo: function() { alert('INFO: ' + this.get('name') + ' in ' + this.get('when')); } });
In this case, added function will be available for all instances of Book model:
myBook.showInfo();
Interaction with the server
Models can be used to represent data stored on the server side, and the actions performed on them will be seen as a REST operations.
Example – creating new user:
var UserModel = Backbone.Model.extend({ urlRoot: '/user', defaults: { name: '', email: '' } }); var user = new Usermodel(); // note: no "id" here var userDetails = { name: 'John', email: 'johndoe@gmail.com' }; // we perform POST /user with data // the server should save the data and return the new "id" user.save(userDetails, { success: function (user) { alert(user.toJSON()); } });
So to create a new user and save his data on the server, we create a new instance, and then call the save() method.
The model is recognized by “id” attribute, so if it is null, Backbone.js will send the POST request to the server (urlRoot). To handle the data on the server side, we can for example node.js or PHP.
Sample data: 1, ‘John’, ‘johndoe@gmail.com
Get the model
When we create object instance with set ID, Backbone.js will perform getting data automatically (urlRoot + ‘/id’).
Example:
var user = new Usermodel({id: 1}); // GET /user/1 // the server should return the id, name and email from the DB user.fetch({ success: function (user) { alert(user.toJSON()); } });
Update the model
The model existing on the server, we can update using PUT method.
Example:
var user = new Usermodel({ id: 1, name: 'John', email: 'johndoe@gmail.com' }); // let's try to update the data on the server // PUT /user/1: `{name: 'Gregory', email: 'gregdoe@gmail.com'}` user.save({name: 'Gregory'}, { success: function (model) { alert(user.toJSON()); } });
Deleting model
We removed attributes. Now how to remove whole model (DELETE /user/id).
Example:
// here we have set the id of the model var user = new Usermodel({ id: 1, name: 'John', email: 'johndoe@gmail.com' }); user.destroy({ success: function () { alert('Destroyed'); } });
Tips and Tricks — model validation before saving:
Following code is an example of adding data validation before saving model.
Person = Backbone.Model.extend({ validate: function(attributes) { if (attributes.age < 0 && attributes.name != "Greg") { return "Don't cheat!"; } }, initialize: function() { alert("Welcome"); this.bind("error", function(model, error) { alert(error); }); } }); var person = new Person; person.set({ name: "Test", age: -1 }); // -> displays error var person = new Person; person.set({ name: "Greg", age: -1 }); // -> ok
Collections — Backbone.Collection
Models vs collections?
What is a collection? It is simply ordered set of models.
Example:
var Photo = Backbone.Model.extend({ initialize: function() { console.log("Nice picture"); } }); var Gallery = Backbone.Collection.extend({ model: Photo });
Examples of use:
Model: Photo — Collection: Gallery
Model: Animal — Collection: Zoo
Example – build data collection:
// model var Song = Backbone.Model.extend({ defaults: { name: "Not specified", artist: "Not specified" }, initialize: function() { console.log("Music!"); } }); // collection var Album = Backbone.Collection.extend({ model: Song }); // populate a collection with data var song1 = new Song({ name: "Song 1", artist: "CodersBand" }); var song2 = new Song({ name: "Testing it!", artist: "Tester" }); var myAlbum = new Album([song1, song2]); console.log(myAlbum.models);
Neat and smart. Our application is quickly taking shape.
That’s all for today.
Backbone.js tutorial — summary
If we are looking for a modern framework, for sure we can consider Backbone.js.
This solution opens up excellent approach of application development, a clear structure as well as the possibility of integration.
In the next part we will focus on Router, Views and other elements necessary to finish basic Backbone.js tutorial for beginners.
Thank you!