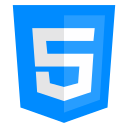
Do you want something great? No problem — in HTML5 we can find a lot of great things. You probably know that HTML5 introduces new elements, but it’s not everything!
We can define HTML5 custom attributes — new, our own attributes, adjusted for our needs.
HTML5 custom attributes
The data-* attributes are used to store our custom data for page (application) elements. We can put them in any HTML elements.
However, we should pay attention to:
– the names of our own HTML5 attributes should start with the “data-” prefix, followed by at least one character,
– the name shouldn’t contain uppercase letters,
– attribute value can be any string.
Syntax:
<element data-*="somevalue">
In JavaScript to our own attribute we refer simply by getAttribute() method.
Practical example should illustrate action of HTML5 attributes defined by ourselves.
Example — using HTML5 custom attributes:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>Testing own attributes in HTML5</title> <script type="application/javascript"> function showCarDetails(car) { var carBrand = car.getAttribute("data-car-brand"); alert("The " + car.innerHTML + " => " + carBrand); } </script> </head> <body> <h1>Cars</h1> <p>Click to see related car brand:</p> <ul style="cursor: pointer; width: 200px;"> <li onclick="showCarDetails(this)" id="Bora" data-car-brand="VW">Bora</li> <li onclick="showCarDetails(this)" id="Cruze" data-car-brand="Chevrolet">Cruze</li> <li onclick="showCarDetails(this)" id="Octavia" data-car-brand="Skoda">Octavia</li> <li onclick="showCarDetails(this)" id="S60" data-car-brand="Volvo">S60</li> </ul> </body> </html>
An on-line example.
best woocommerce composite https://products-wizard.troll-winner.com/ product builder wordpress
Reading the value
Getting the value of an attribute for each element we can perform with a simple call:
car.getAttribute("data-car-brand");
In modern web applications, such capabilities can greatly simplify the programming of various functions, including filtering, sorting, deleting items, etc.
Nay, we can create more advanced apps, and good user experience is achievable without AJAX, because we can keep necessary data as an element’s attribute, available on demand.
Setting values
Setting values for our HTML5 custom attributes is done in the same way, as for other attributes, through the setAttribute() method, as in the following example.
Example — set the value for custom HTML5 attribute:
function showCarDetails(car) { car.setAttribute("data-car-brand", "FooBar"); var carBrand = car.getAttribute("data-car-brand"); alert("The " + car.innerHTML + " => " + carBrand); }
So the modified function displays always “FooBar” as the value.
Many attributes and the dataset shortcut
Can I define multiple attributes for one item? As the most.
Let’s consider:
<span id="dominik" data-birthday="27-03-1986" data-place="Stargard"> This element stores the date and place of my birth. </span>
Example — getting the value in JavaScript:
var dominik = document.getElementById('dominik'); alert(dominik.getAttribute("data-birthday")); alert(dominik.getAttribute("data-place"));
Getting data in this way should work in all browsers. There is also a kind of “shortcut” to the data, named dataset.
Example — getting data from an attribute using dataset:
var dominik = document.getElementById('dominik'); alert(dominik.dataset.birthday); alert(dominik.dataset.place);
Simple.
jQuery
Work with HTML5 custom attributes is supported also by the data() function from jQuery:
https://api.jquery.com/jQuery.data/
For example:
// add an attribute to the element and set default value $('#my-input').data('default-value', 'myDefaultVal'); // set
The .data() method allows to bind the value with an element, e.g. store data for the character in the game:
$("#enemy").data("lifes", 2);
So the handling code from the previous example would look as follows:
var dominik = $('#dominik'); alert(dominik.data('birthday')); // get alert(dominik.data('place')); // get
Simple and effective.
Summary
I hope this short article has given an insight about how brilliant the originators of HTML5 custom attributes were. When I used custom attributes first time, I was really pleasantly surprised.
Thank you for your attention.